CSS, or Cascading Style Sheets, is a stylesheet language used to describe the presentation of a document written in HTML or XML. It allows you to control the layout, formatting, and appearance of multiple web pages all at once.
FORM AND TABLE
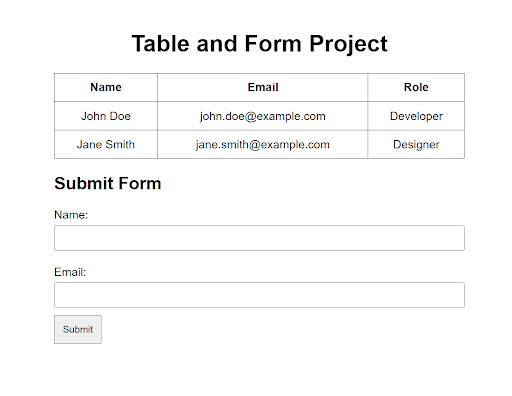
Code step by step:1. Document Type Declaration:
<!DOCTYPE html>
- Declares that the document is an HTML5 document.
2. HTML Opening Tag:
<html lang="en">
- The root element of the HTML document.
- lang="en" specifies that the content is in English.
3. Head Section:
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Table and Form Project</title>
</head>
- Contains metadata about the HTML document.
- <meta charset="UTF-8">: Sets the character set to UTF-8.
- <meta name="viewport" content="width=device-width, initial-scale=1.0">: Configures the viewport for responsive design.
- <title>Table and Form Project</title>: Sets the title of the webpage.
4. Style Section:
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 50px;
}
table {
width: 50%;
margin: 20px auto;
border-collapse: collapse;
}
/* ... Other CSS rules ... */
</style>
- Embeds CSS styles directly into the HTML document.
- Defines styles for body and table elements.
5. Body Opening Tag:
<body>
- Marks the beginning of the HTML body section.
6. Heading and Table:
<h1>Table and Form Project</h1>
<table>
<!-- ... Table Content ... -->
</table>
- <h1>: Defines a top-level heading with the text "Table and Form Project."
- <table>: Defines an HTML table.
7. Table Header and Body:
<thead> <tr>
<th>Name</th>
<th>Email</th>
<th>Role</th>
</tr>
</thead>
<tbody>
<!-- ... Table Rows ... -->
</tbody>
- <thead>: Defines the table header section.
- <tr>: Defines a table row.
- <th>: Defines a table header cell.
- <tbody>: Defines the table body section.
8. Table Rows:
<tr> <td>John Doe</td>
<td>john.doe@example.com</td>
<td>Developer</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>jane.smith@example.com</td>
<td>Designer</td>
</tr>
<!-- Add more rows as needed -->
- <td>: Defines a table data cell.
- Provides sample data for the table.
9. Form Section:
<form>
<!-- ... Form Content ... -->
</form>
- <form>: Defines an HTML form.</form>
10. Form Content:
<h2>Submit Form</h2>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email"
name="email" required>
<!-- Add more form fields as needed -->
<button type="submit">Submit</button>
- <h2>: Adds a subheading "Submit Form" above the form.
- <label>: Labels for form fields.
- <input>: Defines
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
H<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 50px;
}
table {
width: 50%;
margin: 20px auto;
border-collapse: collapse;
}
th, td {
padding: 10px;
border: 1px solid #ddd;
}
form {
width: 50%;
margin: 0 auto;
text-align: left;
}
label {
display: block;
margin-top: 10px;
}
input {
width: 100%;
padding: 8px;
margin-top: 5px;
margin-bottom: 10px;
box-sizing: border-box;
}
button {
padding: 10px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Table and Form Project</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Role</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>john.doe@example.com</td>
<td>Developer</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>jane.smith@example.com</td>
<td>Designer</td>
</tr>
<!-- Add more rows as needed -->
</tbody>
</table>
<form>
<h2>Submit Form</h2>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email"
>Email:</label>
<input type="email" id="email" name="email" required>
<!-- Add more form fields as needed -->
<button type="submit">Submit</button>
</form>
</body>
</html>
Output